In this article, we will learn about different approaches available to implement entity framework in any .NET or .NET Core based C# applications. There are 3 possible approaches when it comes to implementing entity framework in .NET C# applications. These approaches are as follows
- Code First
- Database First
Model First
The entity framework code first is the most preferred approach. The choice you make for the approach to implement entity framework core in your application will influence your overall working experience with entity framework core. If you don’t make the right choice for your application then it will be difficult to manage your application.
In this post, I will not cover how to implement entity framework core in ASP.NET Core. If you want to know about how to implement Entity Framework core in ASP.NET Core, I recommend you also read my other article – Entity Framework Core in ASP.NET Core – Getting Started
Let us understand all the 3 available approaches in detail such that we are able to decide when to use which approach in our application.
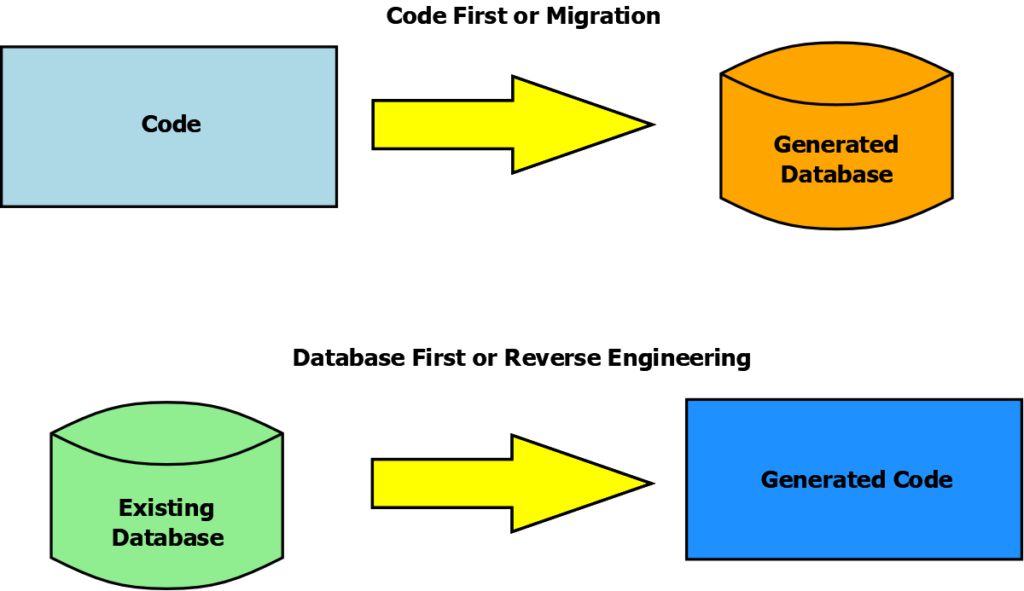
Entity Framework Code First
This entity framework code first approach is the most popular approach for implementing entity framework in .NET C# applications. In this approach as the name suggests instead of working with a database, we create the code first i.e. first we add database entities in our application.
The entity framework will create the database with tables as per the entities classes defined in the application. So code is created first and this code is responsible to create the database using commands available in the EF Core tools package for the creation of the database.
In this entity framework code first approach there won’t be any manual changes to the database as all the changes to the database will be handled through the code. First, you make changes to the code in your applications and then by using the entity framework update-database command you migrate those changes to your database.
There is a number of available data annotation attributes that help you to define foreign keys, constraints, indexes, etc in the C# code.
Since the database is always created or updated from the code so there will be hardly any mismatch between the code and database for columns types, table names, column names, etc. Also, since the database is managed from C# code there is no need for a database administrator to maintain the database.
You normally use this approach for your new applications being developed for the first time (i.e. not a migration application) and that are not database-centric.
Microsoft has started to refer to this approach as Migrations
Entity Framework Database First
This is how we used to develop our applications before entity framework i.e. we used to design database first and then code our application to talk to this database.
You use this approach when you already have a database designed and want to build your application around it i.e. scenario when you want to migrate your existing applications to newer technologies then in that case you have a database already running on production and want to build a new application around this database.
If you already have a designed database then entity framework commands will create the C# database entities from the database design i.e. based on tables, columns, foreign keys and constraints. You will have to make changes to the database manually using scripts and entity framework commands will help you update the model from the database.
We can run commands more than once overtime to get the latest changes from the database into the code. Also, it is not advisable to make changes to code generated by the entity framework as the manual changes you make will be overwritten the next time you command to update your application code as per the database changes.
Microsoft has started to refer to this approach as reverse engineering
Entity Framework Model First
In this approach, you create the model first by using the entity framework designer and this model created in a designer is a definition of database entities in the EDMX file (.edmx extension). This model can be used to generate database schema and also auto-generate C# classes of your application that are used to interact with the database.
This EDMX file can be viewed and modified again using the entity framework designer.
Learn how to choose right approach
Entity Framework Code First – If you have started working on a new application that doesn’t have a database already designed and also if want your entity framework core entities to be the main source of your application then you should entity framework code first or Migrations. Choose this approach so that as and when you make changes to the classes in code you can update the database from the classes in the code.
This approach gives you full control over the code and there won’t be any autogenerated code that is hard to modify and maintain. In this approach, the database is not so important and the database is just some storage to support your application.
Database First – If you are working on a migration project that already has a database available on production or if your application is database-centric then in that case you should use the database first approach or reverse engineering. You can make manual changes to the database and use entity framework commands to update classes in code in your application from the database.
This approach gives you full control over the database. This approach should be preferred when the database is important and contains the business logic of your application.
Summary
We learned about the approaches available for the implementation of entity framework in .NET C# applications. The entity framework code first or migrations is the most preferred approach for newer applications i.e. applications being developed from scratch and database first or reverse-engineering approach should be preferred for applications for which database design is already available.
Hope you enjoyed reading this article, please provide your suggestions & feedback in the comments section below
You can also check my other trending articles on Build Resilient Microservices (Web API) using Polly in ASP.NET Core & Microservices with ASP.NET Core 3.1 – Ultimate Detailed Guide
Hope you found this article useful. Please support the Author
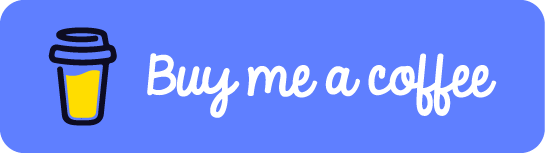