Sometimes when our code is running slow or taking a long time for completion then there is a need to measure the time taken by different code operations (validations, database calls, external API calls, etc.) to identify a slow task that requires improvement in the performance. In this article, we will focus mainly on how to measure elapsed time in C# .NET Core code using Stopwatch so that we are able to determine the time taken by each operation of code.
Measure Elapsed Time in C#
Using System.Diagnostics.Stopwatch Class
To accurately measure the execution time of the code operations we can make use of the Stopwatch class which is part of the System.Diagnostic namespace. This Stopwatch class is almost the implementation or simulation of the real Stopwatch. The Stopwatch class provide methods and properties that are appropriate for accurate measurement of elapsed time for any logical block in the code.
Below is the code that demonstrates how to measure elapsed time using Stopwatch in the console application
using System; using System.Collections.Generic; using System.Diagnostics; using System.Linq; namespace ProCodeGuide.Sample.Async { public class Program { static readonly Stopwatch timer = new Stopwatch(); public static async Task Main(string[] args) { timer.Start(); Console.WriteLine("Program Start - " + timer.Elapsed.ToString()); await PerformValidationsAsync(); Console.WriteLine("Validation Performed - " + timer.Elapsed.ToString()); await GetDataFromAPIAsync(); Console.WriteLine("Got Data from API - " + timer.Elapsed.ToString()); await SaveToDBAsync(); Console.WriteLine("Saved to DB - " + timer.Elapsed.ToString()); timer.Stop(); } private static async Task PerformValidationsAsync() { await Task.Delay(2000); } private static async Task GetDataFromAPIAsync() { await Task.Delay(3000); } private static async Task SaveToDBAsync() { await Task.Delay(5000); } } }
To measure elapsed time in C# .NET you need to call the start method on the stopwatch class at the point from where you need to start measuring the time i.e. click of the button or method start or network call start or database save start point. Next, you will have to call the stop method once you hit the point in the code where you want to stop measuring time and then you can use elapsed property to check the elapsed time between start and stop calls.
As seen from the above code you can also make use of the elapsed property of the stopwatch class even when the stopwatch is running to check the elapsed time till that point of code. When the stopwatch is started the elapsed time will increase gradually till the stopwatch is stopped.
After running the above code we can see the below output in the console window.

Instead of using the Elapsed property of stopwatch which return a TimeSpan you can even make use of ElapsedMilliseconds or ElapsedTicks properties which return elapsed time as Milliseconds or Ticks respectively.
You can format the TimeSpan instance into a text format to print or log it and also you can pass TimeSpan to another method or class that needs an instance of TimeSpan
Don’t use standard DateTime class for measuring execution time as that won’t give accurate results like Stopwatch class
You can make use of the reset method of the stopwatch class to clear the cumulative elapsed time of the existing stopwatch instance. For example, you start to measure elapsed time in the code for a network call once the network call is completed and elapsed time has been logged for that operation then you can reset the stopwatch elapsed time to measure elapsed time for the next logical block of the code i.e. either database call or another network call, etc.
You can format elapsed time as per the Hours, Minutes & Seconds format using the code shown below
Console.WriteLine("Saved to DB - {0:hh\\:mm\\:ss}",timer.Elapsed);
In the above code, we have used the format string to format the elapsed time in hh, mm & ss format. This format is useful for long-running tasks.
Summary
It is this simple to use the Stopwatch class to measure elapsed time (execution time) in C# .NET 6. Also, making use of the Stopwatch class is the most appropriate and accurate way to measure elapsed time in C# .NET
You can even make use of the Now property of the DateTime class to measure elapsed time but that won’t provide an accurate elapsed time as compared to the Stopwatch class.
Please provide your suggestions & questions in the comments section below
You can also check my other trending articles on Build Resilient Microservices (Web API) using Polly in ASP.NET Core & Microservices with ASP.NET Core 3.1 – Ultimate Detailed Guide
Hope you found this article useful. Please support the Author
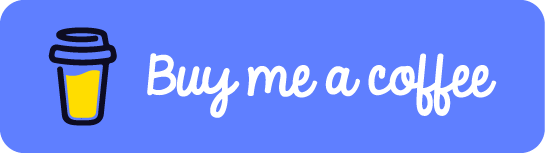
Great share!!
Thanks for your feedback!