In this article, we will learn mainly about what are string, String & StringBuilder in C# their differences and when to use them. String vs StringBuilder details will help you to decide what should be used in your code for better performance and memory management.
Table of Contents
C# string or String
A string (small s) or System.String is a sequential collection of characters that is used to store text in C#. The string is immutable means it is read-only i.e. if you attempt to modify a string then instead of updating the value it will create a new object of string in memory. A string can hold a maximum of 1 billion characters which is approximately 2GB in size in memory.
Below is the example of string or String types
string FirstName = "Pro"; String LastName = new String("Code Guide"); FirstName = "Pro Code Guide"; //Will create new object in memory
Let’s first try and understand the difference between the string (small s) and System.String data types in C# .NET
string vs System.String
There is no difference between string & String (with capital S) in C#. System.String (String with capital S) is a class in C# in the System namespace. string (with small s) is an alias of System.String
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace ProCodeGuide.Sample.Async { public class Program { public static void Main(string[] args) { string FirstName = "Pro"; String LastName = new String("Coder"); Console.WriteLine("First Name - " + FirstName); Console.WriteLine("Last Name - " + LastName); Console.WriteLine(FirstName.GetType().FullName); Console.WriteLine(LastName.GetType().FullName); } } }
In the above code, we have declared a couple of variables (FristName & LastName) of type string but Firstname is declared as a string (with small s) and LastName is declared as System.String. Both variables have been assigned values upon declaration and then we have printed values as well types of both the variables.
After running the above code we can see the below output in the console window.

We can see from the above output that both string & String are of the same type. You can use any of the 2 but the most popular preferred is a string (with small s). Most developers including me use strings to declare variables in C#
C# StringBuilder
In C# string type is immutable whereas StringBuilder is a mutable string of characters means its value can be changed without creating a new object in memory on each modification.
From a performance point of view, StringBuilder is always preferred over string in scenarios where your program requires performing some heavy string manipulations like modifying values or string concatenations. As when there are repetitive operations happening on the same string then in that case if you use string or System.String then it will create a new object on each operation and slow down the performance of the application and also end up using more memory on the heap.
Below is the example of string or StringBuilder types
using System.Text; // include this namespace StringBuilder LastName = new StringBuilder("Pro"); LastName.Append(" Guide"); //Will append String to StringBuilder and will not create new object in memory string LastNameStr = LastName.ToString(); //To access string in StringBuilder LastName.Insert(4," Code"); //LastName = Pro Code Guide LastName.Remove(4, 5); //LastName = Pro Guide LastName.Replace("Guide", "Code Guide"); //LastName = Pro Code Guide
The above code shows how to instantiate an object of type StringBuilder and also how to perform various operations on StringBuilder object like assign value to StringBuilder, Append the string to StringBuilder, access string in StringBuilder, Insert string into StringBuilder, Remove string from StringBuilder and Replace string in StringBuilder.
String vs StringBuilder
When it comes to string vs StringBuilder both represent a collection of characters but StringBuilder is a mutable (can be modified) string class i.e. it can be modified by appending, removing, inserting or replacing.
Any modification of a string will create a new object in memory on each modification so don’t use string where you will modify a string numerous times in a loop as that can lead to significant memory utilization of the application. Use StringBuilder When you expect your code to make a significant number of modifications to a string.
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace ProCodeGuide.Sample.Async { public class Program { public static void Main(string[] args) { string FirstName = "Pro"; StringBuilder LastName = new StringBuilder("Code"); LastName.Append(" Guide"); Console.WriteLine("First Name - " + FirstName); Console.WriteLine("Last Name - " + LastName.ToString()); Console.WriteLine(FirstName.GetType().FullName); Console.WriteLine(LastName.GetType().FullName); } } }
After running the above code we can see the below output in the console window.

I hope after reading you got a fair idea of string vs System.String vs StringBuilder and now you will be able to effectively decide when to use string or StringBuilder in your code.
Check for Empty StringBuilder
The length property of the StringBuilder class can be used to check if the StringBuilder is empty or not. If StringBuilder is empty then the length will be 0
StringBuilder LastName = new StringBuilder("Code"); if(LastName.Length == 0) { Console.WriteLine("LastName is Empty"); }
We can even make use of methods from the String class to check if the StringBuilder is empty or not
StringBuilder LastName = new StringBuilder("Code"); if(string.IsNullOrEmpty(LastName.ToString())) { Console.WriteLine("LastName is Empty"); }
Methods in StringBuilder
StringBuilder supports a variety of methods that can be used to modify or manipulate the string in the StringBuilder class. Here is the list of methods with their use available in StringBuilder for string manipulations
Append() – As the name suggests this method append is used to add a new string value at the end of the current string value in the StringBuilder. You use this method when you need to add some string at the end of the existing string.
AppendLine() – This method like Append() method will add a new string value at the end of the current string value in the StringBuilder but it will add the new string on a new line. You can use this method when you need to add a line terminator to the existing StringBuilder value before adding the new string to the StringBuilder
AppendFormat() – As the name suggests this function allows you to add a string in the required format at the end of the current string value in the StringBuilder. You can use this method when you need to format the string before adding it to the StringBuilder.
Insert() – This method is used to add a new string to the current string value in the StringBuilder at the specified location instead of adding it at the end or new line of the current string. You can use this method when you need to add a string at the start or in the middle of the current string value in StringBuilder.
Replace() – As the name suggests this method is used to replace the occurrences of some string in the StringBuilder with the new string value specified in the replace method. If you specify the empty value for the new string in the replace method then simply the occurrences of some string in the StringBuilder will be removed.
Remove() – The remove method is used to remove the specified number of characters, starting from the specified location, from the string in the StringBuilder. You can use this method when you know the string pattern and need to remove the specified number of characters starting at the specified location.
Clear() – This method is used to remove all the characters from the string value in the StringBuilder.
ToString() – This method converts the value of the StringBuilder to a string.
Compare String vs StringBuilder Performance (StringBuilder Performance)
To measure & compare the performance difference between String & StringBuilder types we will write a small program that first creates a list of strings and we will populate this list of stings with generated numbers.
Then we will traverse this list using a foreach loop and concatenate each value in the list to form one large string. Now we will perform this concatenation twice as in one loop we will use string type and in the second loop, we will use StringBuilder type. The code for this operation is shown below
public class Program { static readonly Stopwatch timer = new Stopwatch(); public static void Main(string[] args) { timer.Start(); List<string> ListofNumbers = Enumerable.Range(1, 100000) .Select(number => number.ToString()).ToList(); Console.WriteLine("List Prepared - " + timer.Elapsed.ToString()); String finalResult = ""; foreach (String strvalue in ListofNumbers) { finalResult += strvalue; } Console.WriteLine(finalResult.GetHashCode()); Console.WriteLine("Loop with Type String Completed - " + timer.Elapsed.ToString()); StringBuilder sb = new(); finalResult = ""; foreach (String strvalue in ListofNumbers) { sb.Append(strvalue); } finalResult = sb.ToString(); Console.WriteLine(finalResult.GetHashCode()); Console.WriteLine("Loop with Type StringBuilder Completed - " + timer.Elapsed.ToString()); } }
We have made use of the Stopwatch class to measure the time taken by each operation. After running the above code we will get the output as shown below
List Prepared - 00:00:00.0671332
30470787
Loop with Type String Completed - 00:00:25.1349235
30470787
Loop with Type StringBuilder Completed - 00:00:25.1374292
As you can see from the above results concatenation with String type took around 25 secs whereas with StringBuilder it just took a few milliseconds. So performance-wise if you are doing any heavy string operations or continuous string operations in a loop then it is always recommended to StringBuilder for better results.
Also while running the above code in Visual Studio you can also check in the diagnostics windows is that process memory is also high when the loop with String type is executing as String is immutable and it will always create a new object in memory.
Summary
In this article, we learned about what is C# StringBuilder and the differences between string vs StringBuilder with various examples. We also looked at strings and string (with small s) vs System.String.
I hope you enjoyed this post and found it useful. If you have any doubt, please feel free to post your questions or suggestion in the comment section.
Hope you enjoyed reading this article, please provide your suggestions & feedback in the comments section below
You can also check my other trending articles on Build Resilient Microservices (Web API) using Polly in ASP.NET Core & Microservices with ASP.NET Core 3.1 – Ultimate Detailed Guide
Hope you found this article useful. Please support the Author
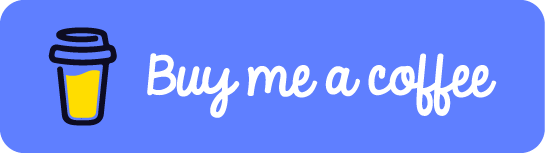
There is one subtle difference between “string” and “String”. “String” requires you to include “using System;”, while “string”, being an alias for the fully-qualified name, does not. Of course, 99.9% of the time, you’ll be including “using System;” anyway, but one should recognize that as one of the side-effects of forgetting to.
Agree..Thanks