Encapsulation is one of the important building blocks in object-oriented programming. It is a mechanism in which data and code which manipulate this data are enclosed into a single unit. This unit can be a class in c# which allows the developer to hide data by making variables inaccessible outside the class and providing functions, which can be accessed outside this class, to access these variables.
The idea is to hide the internals of an object behind specific methods i.e. restrict the direct access to some of an object’s components. In c# class, there are properties that allow class variables to be exposed by using get and set accessors.

As you can see in the above code, in the Employee class its private data member Name is exposed by using public property Name with set and get accessors. Set is used to change the value of data members and Get is used to read the value of data members. Also, validation rules if any can be implemented in the set method when the value is being changed to ensure that the new value is within desired limits. This way you can control access to the internal state of the object.
In encapsulation one single unit i.e. class is formed with data members that store the current state of the object and the method that can be used to access these data members.
Access modifiers & access levels
Encapsulation is implemented by using access specifiers. All classes and class members can specify what access level they provide to other classes by using access modifiers. Only one modifier can be used per class, method & data member. An access specifier specifies the scope and visibility of class members.
Modifier | Definition |
---|---|
public | The type or member can be accessed by any other code in the same assembly or another assembly that references it. |
private | The type or member can only be accessed by code in the same class. |
protected | The type or member can only be accessed by code in the same class or in a derived class. |
internal | The type or member can be accessed by any code in the same assembly, but not from another assembly. |
protected internal | The type or member can be accessed by any code in the same assembly, or by any derived class in another assembly. |
private protected | The type or member can be accessed by code in the same class or in a derived class within the base class assembly. |
Encapsulation – c# .NET example
Here is an example of an encapsulated class Employee in which we have specified properties Name & Total Experience to be specified by User. Employee Id is generated internally in class and generation logic is not exposed to the user also user can only read Employee Id and cannot change it. Similarly, salary is also calculated internally in class, and the user can access employee salary by the GetSalary method.
class Employee { private string name; private long id; private double yearsofexperience; public Employee() { id = EmployeeService.GetEmployeeId(); } public long Id { get { return id; } } public string Name { get { return name; } set { name = value; } } public double YearsOfExperience { get { return yearsofexperience; } set { yearsofexperience = value; } } public double GetEmployeeSalary() { double salary = 0; if (yearsofexperience == 0) salary = 50000; else if (yearsofexperience <= 5) salary = yearsofexperience * 100000; else if (yearsofexperience > 5) salary = yearsofexperience * 1.5 * 100000; return salary; } }
In the below example, the Employee class has been used and by specifying the Name & Experience of the Employee user is able to get Id & salary without worrying much about the internals of the Employee class
public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void CreateEmployee() { Employee employee = new Employee(); employee.Name = txtEmployeeName.Text; employee.YearsOfExperience = Convert.ToInt32(txtEmployeeSalary.Text); txtEmployeeId.Text = employee.Id; txtEmployeeSalary.Text = employee.GetSalary().ToString(); } private void btnCreateEmployee_Click(object sender, EventArgs e) { CreateEmployee(); } }
Advantages of Encapsulation
- The internal implementation of the class is hidden from the user of the class. The user is exposed to just specific methods of the class.
- It provides control over the data by allowing developers based on their requirements to consciously provide set or get methods for a private data member.
- It makes it easier to perform unit testing of the class.
Summary
Encapsulation is a fundamental block of object-oriented programming and it prevents access to implementation details. Encapsulation enables the programmer to implement the desired requirement for the level of access to the internals of the object.
It not only allows the programmer to control which attributes can be accessed or modified but also allows to limit changes as per the requirements.
You can also check my other Article explaining ASP.NET Core caching – https://procodeguide.com/programming/aspnet-core-caching/
Hope you found this article useful. Please support the Author
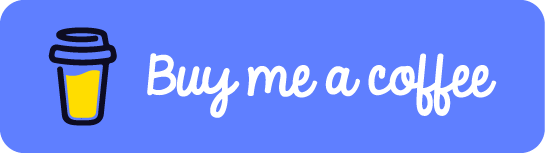