In this article, we will learn about how to upload a file using File Upload Control in ASP.NET C#. We will create a new project of type in ASP.NET C# to develop a solution that will make use of file control to upload a file using File control in ASP.NET C#
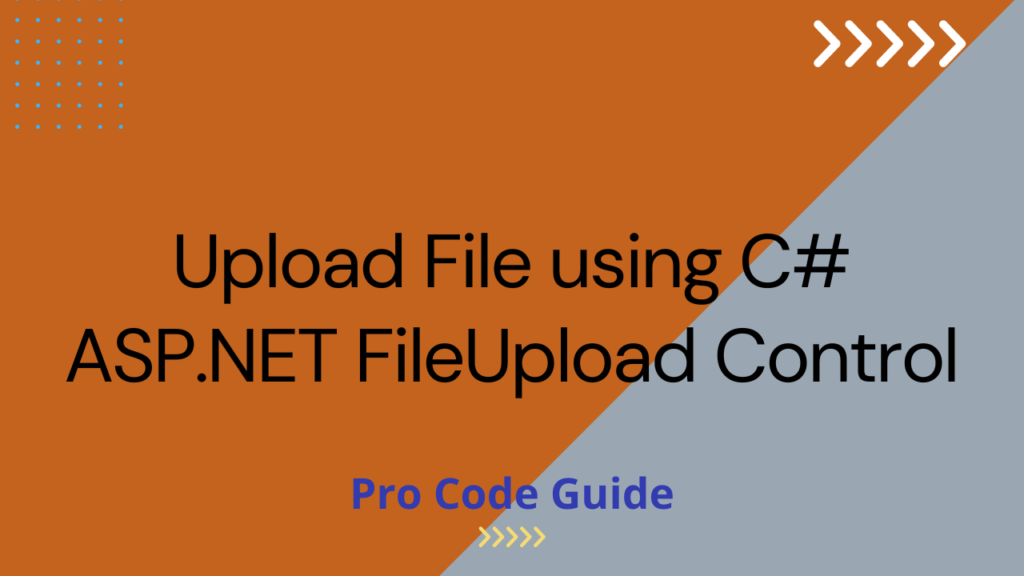
ASP.NET FileUpload control is an ASP.NET Web control that allows the implementation of the functionality of the file upload in ASP.NET web applications. ASP.NET FileUpload control automatically sets the encoding of the web form so there is no need to explicitly set it to “multipart/form-data” in the web form.
Table of Contents
Implement Upload File using C# ASP.NET FileUpload Control
Here is the solution to upload a file using ASP.NET FileUpload control in C#.
Create a New ASP.NET C# project
- Open Visual Studio & select create a new project option
- Select the type of project as ASP.NET Web Application (.NET Framework) and then click on the Next button
- Specify the project name as CodeHandBook.Sample.FileUpload, select the location of the project where it will be saved, and let the solution name be the same as the project name, set the target .NET framework to 4.8 and then click on Create button.
- Specify the type of application as Empty & select Add Folders and core reference as Web Forms and next click on Create button.
- Add the aspx page named “FileUploadForm.aspx” to the project, this web form will be used to upload a file using FileControl in the ASP.NET C# application.
Add FileUpload Control to the form
Add ASP.NET FileUpload control to the the FileUploadForm.aspx page by adding the below line within the <form> tag.
<asp:FileUpload ID="FileUploadControl" runat="server" />
Add button to handle File Upload
To support file upload from the ASP.NET FileUpload control we need to add a button that will upload the file to the server. Add below code for the button within the <form> tag
<asp:Button ID="btnUpload" runat="server" OnClick="btnUpload_Click" Text="Upload File"/>
Add button click handler in the code behind
To receive a file on the server and save the received file to the folder on the server we need to implement the button click in the code behind that will be executed on the server side. Add below code to the FileUploadForm.aspx.cs file for handling the button click event
protected void btnUpload_Click(object sender, EventArgs e) { if (FileUploadControl.HasFile) try { //In SaveAs you need to specify the path where you want to save the file on server FileUploadControl.SaveAs(@"C:\Users\suppo\Documents\" + FileUploadControl.FileName); lblMessage.Text = "File Uploaded Sucessfully !!"; } catch (Exception ex) { //Log Exception Message lblMessage.Text = "File Upload Failed!!"; } else { lblMessage.Text = "Please Select File to Upload"; } }
The above code checks if the file is present in the request and if the file has been sent then it saves the file to the specified folder using the SaveAs method of the FileUpload control and sends a success message to the user. In the above code if no file is present in the request then in that case the code returns an error message saying that the File upload failed.
After running the above code try to upload a file and verify whether the user is able to select & submit a file from the local system. The file uploaded to the server will be saved in the folder specified in the application.
Uploading Large Files using ASP.NET FileUpload Control
In ASP.NET .NET Framework-based applications by default, the maximum upload size is set to 4096 KB (4 MB).
To increase or override this limit you need this limit you will have to add the required section in the application’s web.config file and specify the increased limit for the upload file size.
To specify the file maximum upload size in the web.config of your web application add the below entry in the web.config file
<configuration> <system.web> <httpRuntime targetFramework="4.8" maxRequestLength="214748364" /> </system.web> </configuration>
Restrict File Types in ASP.NET FileUpload Control in C#
If there is a requirement to restrict file upload for certain types of types for example If you are allowing upload for a user profile picture in your ASP.NET web application then you would want to restrict the file type upload to an image file like *.jpg, *.png, etc. As user should be allowed to upload a PDF file for a user profile picture.
Restricting the file type in ASP.NET FileUpload control for upload in your application is also important from a security point of view as this restricts users from uploading an executable or scripts that will allow users to exploit the application.
To control the file types that are allowed to be uploaded in the ASP.NET web application using ASP.NET FileUpload control you need to add a Regular expression validator code in your aspx page that holds the FileUpload control.
Say for example I want to restrict the file upload to types JPG and PNG then for that I will add the below code to the aspx page that allows only JPGs and PNGs to be uploaded in ASP.NET C# web application.
<asp:RegularExpressionValidator id="FileUpLoadValidator" runat="server" ErrorMessage="Only jpg & png are allowed for uploads" ValidationExpression="^(([a-zA-Z]:)|(\\{2}\w+)$?)(\\(\w[\w].*))(.jpg|.JPG|.png|.PNG)$" ControlToValidate="FileUploadControl"> </asp:RegularExpressionValidator>
The above code adds validation for ASP.NET FileUpload control to allow only the jpg & png file types for upload. In the parameter ControlToValidate, you need to specify the ID of the FileUpload control used in your web form.
Also, note that for this Regular Expression validation to work you need to ensure that the below configuration exists in your web.config file
<appSettings> <add key="ValidationSettings:UnobtrusiveValidationMode" value="None" /> </appSettings>
The above configuration will enable the built-in validator controls to use unobtrusive JavaScript for client-side validation logic. The value has been set to None so the ASP.NET C# application will use the pre-4.5 behaviour for client-side validation logic.
After adding the above code when you try to select any file type other than JPG & PNG for upload then it will display a validation message configured in the ErrorMessage parameter in the RegularExpressionValidator code. In this case, it will display “Only jpg & png are allowed for uploads”
Summary
We saw how we could upload a file using ASP.NET FileUpload Control using C# in a web application. You can use this feature of file upload in ASP.NET C# to upload files of any type like pictures, PDF, Excel data files, XML, etc.
Please provide your suggestions & feedback in the comments section below
References – Upload a file to a Web Site
You can also check my other trending articles on .NET Core to learn more about developing .NET Core Applications
- Microsoft Feature Management – Feature Flags in ASP.NET Core C# – Detailed Guide
- Microservices with ASP.NET Core 3.1 – Ultimate Detailed Guide
- Entity Framework Core in ASP.NET Core 3.1 – Getting Started
- Series: ASP.NET Core Security – Ultimate Guide
- ML.NET – Machine Learning with .NET Core – Beginner’s Guide
- Real-time Web Applications with SignalR in ASP.NET Core 3.1
- Repository Pattern in ASP.NET Core with Adapter Pattern
- Creating an Async Web API with ASP.NET Core – Detailed Guide
- Build Resilient Microservices (Web API) using Polly in ASP.NET Core
Hope you found this article useful. Please support the Author
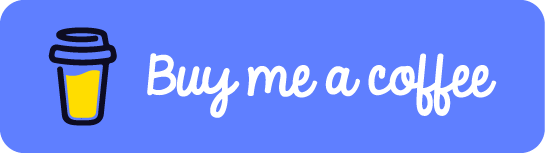
Thanks, this helped me to correct a issue in my code.