In this article, we will discuss the top 10 essential NuGet libraries for ASP.NET Core which every developer should know to develop better ASP.NET Core applications and also reduce the time required to develop these applications.
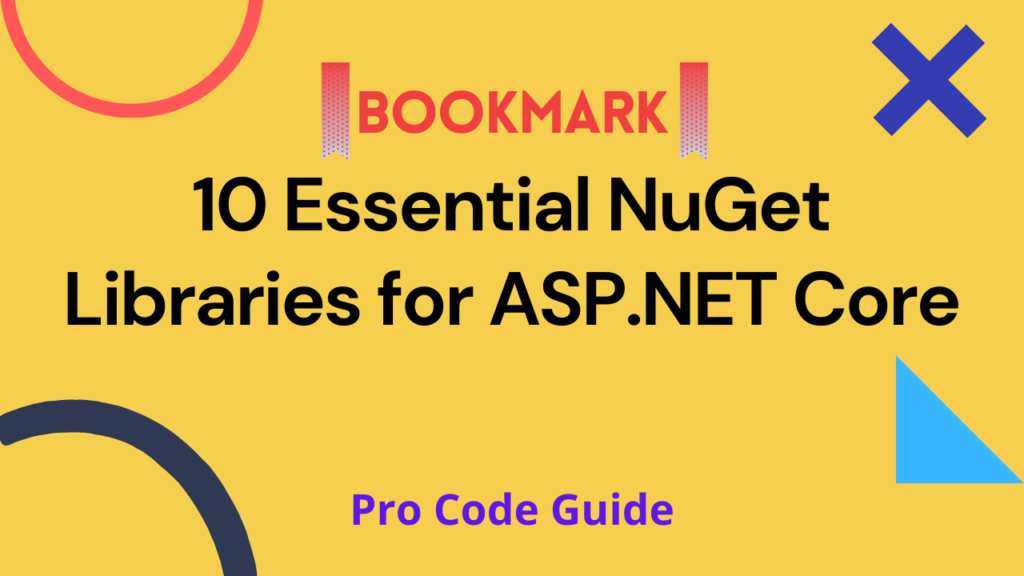
Table of Contents
Overview
The .NET Core framework has grown so rapidly from .NET Core 1.0 to .NET 6 and many libraries also got developed to help developers implement the common functionality required in the application. So it becomes very important for developers to understand which are these libraries and what problems are addressed by using these libraries.
These 10 essential NuGet libraries for ASP.NET Core are free & open-source reusable code written by someone else. The purpose of these NuGet libraries is to help solve the common problems in easier ways and also allow you to improve the productivity of the application development.
Using these libraries will allow developers to use tried and tested code for most of the common features required in your application. This will not only aid in rapid application development but will also allow developers to focus only on the functional requirements of the application.
Pros & Cons of using Third-Party Libraries
It is also important to know the pros and cons of using any third-party library in your application so that you are able to make a well-informed decision. Here are the advantages & disadvantages of using a third-party library in your application
Pros
- Saves time in development & testing
- Developers can focus on functional requirements
- Tried & tested code
Cons
- Dependency on third-party code
- Sometimes there is not enough support available for the third-part libraries
- There might be security issues with the library.
10 Essential NuGet Libraries for ASP.NET Core
Here are the top 10 essential NuGet Libraries for ASP.NET Core to improve the developer’s productivity and develop better applications using these libraries.
Serilog
Application log writing is critical to any application as it allows you to analyse the application behaviour in the production environment and helps you to identify the root cause in case of exceptions occurred in the application. Logging can also be used to log performance parameters to identify the slow running pieces of code. Serilog can be used to implement logging in ASP.NET Core applications.
Serilog is a third-party library that provides functionality for logging application events, information and exceptions to the desired destination like console, file, database, cloud, etc. Serilog also allows the log entries to be formatted as structured logs so that those entries can be read and processed by a computer program.
To know more details on how to implement Serilog in ASP.NET Core you can read my article Setting up Serilog in ASP.NET Core – Detailed Beginner Guide
Library Link – https://github.com/serilog/serilog
Dapper
The object-relational mapping (ORM) is the programming technique in which you write the database (SQL) queries in your preferred language and get the output from the database as the programming language objects. The ORM hides all the complexities and encapsulates the SQL queries into objects. The Dapper is one of the best ORMs that can be used in ASP.NET Core to interact with the database.
A Dapper is a simple object-relational mapper or a micro ORM that can be used for mapping the database results to the .NET C# Objects. By using Dapper in ASP.NET Core we can write SQL statements as if we are writing them in SQL Server management studio but results are mapped to the .NET C# 0domain class object. Dapper provides this interface with the database with good performance results and is almost as fast as RAW ADO.NET. Dapper also supports parameterized queries which can be used to avoid SQL injection attacks on the ASP.NET Core application.
Library Link – https://github.com/DapperLib/Dapper
xUnit
It is always critical to test the application before we deploy it on production or deliver it to the customer for testing as good testing at the developer end ensures good quality of the application being delivered. As the size of the code increases it adds complexity to the application so the time required for testing increases. So for this purpose, it is always better to automate the testing in the application as this will reduce the time & effort required to test the application.
There are applications in some organizations which need to deploy changes to production every hour so automated unit tests in these projects become a necessity to ensure the good quality of the code being delivered. The xUnit.NET can be used to automate unit testing of the application in ASP.NET Core
xUnit.net is a free, open-source, community-focused unit testing tool for the .NET Core & .NET. xUnit.Net is the newest unit testing framework for ASP.NET Core projects and gained popularity when Microsoft started using it to build the code for ASP.NET Core Framework. xUnit.net can be used to implement unit testing in both .NET Core & .NET Full framework as well. XUnit.net is the latest testing framework that implements a set of attributes or decorators that can be used in testing methods and using which we can write unit testing code to autotest software components or units of an application in ASP.NET Core.
To know more details on how to implement Unit Testing in ASP.NET Core you can read my article Implement Unit Testing in ASP.NET Core 5 Application – Getting Started
Library Link – https://github.com/xunit/xunit
Moq
As discussed above automated unit tests are important to deliver a quality product but sometimes writing these unit tests is a difficult task as components being tested might be dependent on other parts and these other parts may not be ready or it might be difficult to use these other parts to write the unit tests. This is where the mock objects come to help, a mock version of an object is something that implements the same interface as the real object so that it can act like a real object but the behaviour of the mock object in the unit test code can be controlled using the configuration or through code.
The mock objects are nothing but fake classes that returns a known and configured value for the input provided. Moq can be used to implement these mock objects for unit testing purposes.
Moq is the most popular and friendly mocking framework available on GitHub for creating mock objects in .NET & .NET Core. Moq supports the configuration of mock objects like you can set up object properties, add methods with input as to specific arguments and return specific values, etc. These mock objects can be used in test code for calls to mock objects.
Library Link – https://github.com/moq/moq
AutoMapper
In your applications where you are interfacing with a database to save data, you will have different objects for view models and database entities. The view model object is used to get data from users and database entities are an exact match with database tables designed to interact with the database. In this scenario, you will have to copy data from the instance of the model to the instance of the database entity.
Since objects are of different classes you cannot set an instance of one object to another object, you will have to use the traditional approach of copying data from one object to another field by field. To simplify this job of mapping data from one object to another object AutoMapper can be used.
Automapper in ASP.NET Core is a small & popular library for an object to object mapping used to map objects of different classes. Automapper in ASP.NET Core is an open-source library and It maps the properties of two different objects. Automapper can be used to map objects of any class but the names of the properties in both the classes should be identical. If the name of the properties is not identical then in that case Automapper provide an option to manually specify the set of source and destination property name.
To know more details on how to implement Automapper in ASP.NET Core you can read my article Implement Automapper in ASP.NET Core 3.1 – Quick & Easy Guide
Library Link – https://github.com/AutoMapper/AutoMapper
Newtonsoft Json.NET
These days it is very common for applications to make calls to Web API for various reasons like data retrieval, email service, SMS service, cloud service, etc.. These APIs can be our own developed projects or other third party API. When it comes to exchanging data between different APIs JSON is the most popular & preferred format. When you are working on ASP.NET Core applications & interacting with Web API then JSON data string conversion to .NET C# object and vice versa will be required to access JSON based API.
The process of converting the .NET C# objects into JSON format strings is known as serialization and the conversion of JSON format strings into .NET C# objects is known as deserialization. Newtonsoft Json.NET is a library than can be used for serialization and deserialization.
Json.NET is a JSON framework for .NET from Newtonsoft. Json.NET is a very popular and high-performance framework for JSON. Json.NET supports features like Serialization and deserialization of any .NET C# object, LINQ to Json, XML Support, etc. Newtonsoft Json.NET is high performance & easy use third party library. Json.NET supports multi-platforms like Windows, macOS, Linux, Mono & Xamarin.
Library Link – https://github.com/JamesNK/Newtonsoft.Json
FluentValidation
Today we are writing public APIs that can be accessed by anyone on the internet and different users can provide all sorts of input. Today someone with the wrong intent can bring down our application if we don’t validate the input properly. So the input data validations are critical for any application to function without any issues due to the different input provided by the users.
When it comes to input validation in ASP.NET Core C# the most preferred approach is Data annotations in which you specify attributes for each property in the model. These attributes are the validations that get performed when the model is validated for processing. This approach is not extensible and also model can get bloated. Also, these data annotations in the model are not in line with the clean code architecture and SOLID principles. The FluentValidation library can be used to perform validation in ASP.NET Core to address some of the concerns.
FluentValidation is a free and popular .NET validation library than can be used to build strongly-types validation rules. The validations rules in FluentVlidations are easy to create, clean and maintain. It doesn’t bloat your model like data annotations. By using FluentValidations for validations in ASP.NET Core you have better control over the validations in your application.
Library Link – https://github.com/FluentValidation/FluentValidation
Polly
We will be not fully correct if we assume that we have thoroughly tested our application and so there won’t be any outages in the production environment. Application failures are bound to happen in the production environment. These application failures can be due to unforeseen reasons like high loads, hardware failure, network issues, third-party API failures, etc.
Also in today’s Microservices world, it is required to create fault-tolerant or resilient applications i.e. applications where service is always available with acceptable functionality and performance even in conditions like high load, third party service failures, network failures, etc. Polly can be used to build resilient web services or microservices in ASP.NET Core.
Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner.
By implementing Polly in ASP.NET Core we can build resilient applications that can be designed to function smoothly in spite of partial failure that occur in the application. With Polly, just a few lines of code are required to implement the Fault Tolerance in Microservices. Polly supports policies like Retry, Circuit Breaker, Timeout, Bulkhead Isolation, Cache, Fallback & Policy Wrap.
To know more details on how to build resilient applications with Polly in ASP.NET Core you can read my article Build Resilient Microservices (Web API) using Polly in ASP.NET Core
Library Link – https://github.com/App-vNext/Polly
Swashbuckle
When you have developed a Web API that will be consumed by different other applications than in that case providing full detailed documentation for API is very important. Also, developers consuming this web API are trying to resolve business issues so understanding its various methods is very important. The API documentation provides instructions about how to use and integrate API into your application. This problem of quickly & accurately generating full detailed useful documentation for the web API can be addressed by Swagger that is also known as OpenAPI.
Swashbuckle is an open-source library to generate swagger documents for ASP.NET Core Web API. You can generate beautiful API documentation, including a UI to explore & test API action methods. It provides features such as client SDK generation, interactive documentation & API discoverability. Swashbuckle also provides awesome swagger-ui that is powered by the generated swagger JSON.
Swashbuckle mainly provides 3 main features Swagger object model & middleware to expose swagger documents as the endpoint, functionality to generate swagger JSON documents and an embedded version of swagger-ui tools.
Library Link – https://github.com/domaindrivendev/Swashbuckle.AspNetCore
Health Checks
In today’s digital world availability of the applications is critical for the business to function. If your application is down then it leads to a loss of revenue, unhappy customers & have an impact on business reputation. From the hardware side, we take many steps to avoid failure like load-balanced servers, failover sites, disaster recovery sites, disk mirrors, etc. What if our application is unhealthy, crashed or not functioning properly.
So we need to proactively check the health of our application i.e. we need to detect the errors, if any, in our application before the users start facing problems and report the same. Using the health libraries available in ASP.NET Core we can determine the unhealthy application and take the action to fix it immediately or inform the customer about the outage with the time required for resolution.
The library for health checks in ASP.NET Core allows for configuring checks that can report the health of the application. These health checks can be exposed as HTTP endpoints in any ASP.NET Core application. This library can be used to configure application health checks like memory, disk, server resources & other application dependencies like database or third party service endpoints.
Library Link – https://www.nuget.org/packages/Microsoft.Extensions.Diagnostics.HealthChecks/6.0.5
Summary
In this article, we learned about which are these 10 essential libraries for ASP.NET Core, why should we use these libraries & what features are offered by these libraries? Using these libraries in your project will allow you to use tried and tested code for common functionalities & you can focus only on the business functionalities that need to be developed as part of your application.
Please provide your suggestions & questions in the comments section below
You can also check my other trending articles on .NET Core to learn more about developing .NET Core Applications
- Microsoft Feature Management – Feature Flags in ASP.NET Core C# – Detailed Guide
- Microservices with ASP.NET Core 3.1 – Ultimate Detailed Guide
- Entity Framework Core in ASP.NET Core 3.1 – Getting Started
- Series: ASP.NET Core Security – Ultimate Guide
- ML.NET – Machine Learning with .NET Core – Beginner’s Guide
- Real-time Web Applications with SignalR in ASP.NET Core 3.1
- Repository Pattern in ASP.NET Core with Adapter Pattern
- Creating an Async Web API with ASP.NET Core – Detailed Guide
- Build Resilient Microservices (Web API) using Polly in ASP.NET Core
Hope you found this article useful. Please support the Author
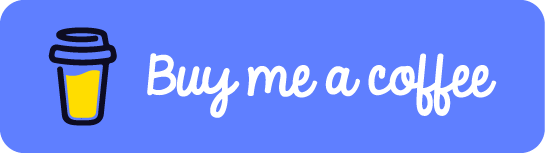