Let’s learn about Python boolean data type.
In Python programming language boolean refers to values either True or False. When you compare anything then the expression is evaluated and the result is either True or False so the boolean data type can be used to evaluate comparison-related expressions.
Here is the source code to demonstrate Python boolean data type
print(50 > 5) print(50 == 5) print(50 < 5) var1 = 50 var2 = 5 if var2 > var1: print("var2 is greater than var1") else: print("var2 is not greater than var1")
Below is the output of the above code
True
False
False
var2 is not greater than var1
As you can see from the above output the evaluation of the comparison expression in the print() function or in the if statement returns either True or False.
Python bool() Function
In Python programming language the Python bool() function can be used to evaluate any value or variable. The evaluation of any value using the bool() function returns either True or False.
Here is the source code to demonstrate the Python bool() function
print(bool("Python")) print(bool(250)) var1 = "Python" var2 = 250 print(bool(var1)) print(bool(var2))
Below is the output of the above code
True
True
True
True
As you can see from the above output the bool() function evaluates the value or variable and returns True.
Most of the values in the bool function will be evaluated as True i.e. any string, number, array, list, tuple, set and the dictionary will evaluate as True but there are some values that will evaluate as false as well like the empty string, 0 and empty list, set, tuple, etc.
print(bool(False)) print(bool(None)) print(bool(0)) print(bool("")) print(bool(())) print(bool([])) print(bool({}))
Below is the output of the above code
False
False
False
False
False
False
False
As you can see from the above output the bool value False, number 0 & empty values have been evaluated as False.
Summary
You can also check my series on Python for Beginners – Series: Python Tutorial – Learn Python Programming for Beginners
References – Python Documentation
Please provide your suggestions & feedback in the comments section below
Hope you found this article useful. Please support the Author
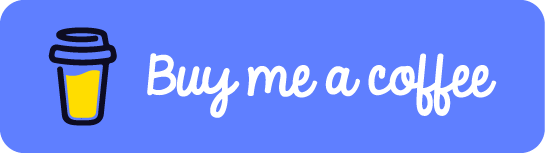