In this article, we will learn about Python string data types. We will explore how to assign text or multiple-line text to a string in Python programming language. We also learn about how to read data in string and perform various operations on string data type
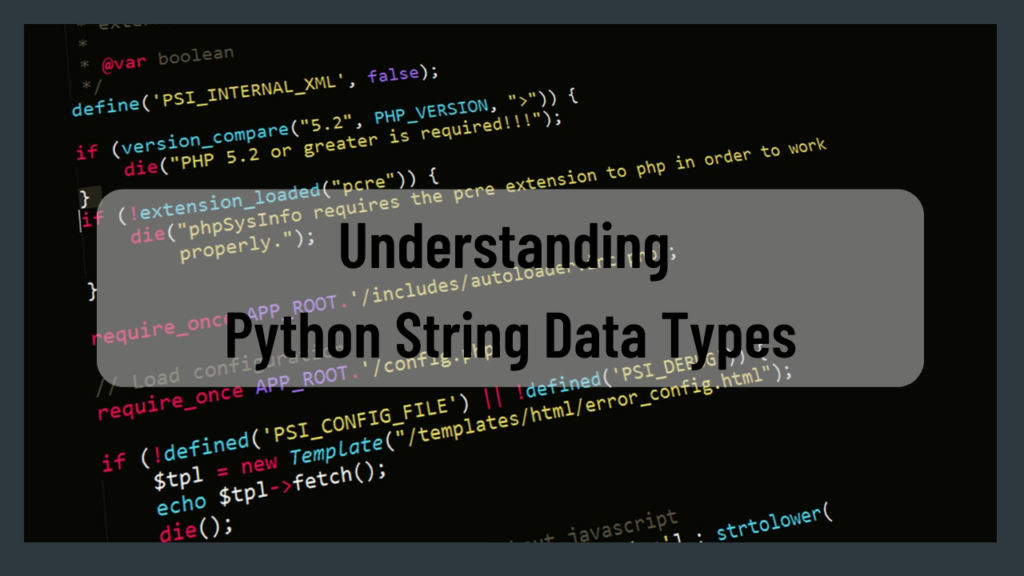
Table of Contents
Introduction to Python String Data Type
Strings are the most popular data type with developers some new developers even end up storing numbers as strings. Python strings data type can be created by enclosing the sequence of characters in single or double quotes i.e. ‘Hello, World!’ or “Hello, World!”.
Here is the source code to demonstrate the use of Python strings data type
message = 'Hello, World!' messagenew = "Hello, World!" print(message) print(messagenew) print(type(message)) print(type(messagenew))
Below is the output of the above code
Hello, World!
Hello, World!
<class 'str'>
<class 'str'>
As you can see from the above output both the variables message & messagenew are of type str (string) and there is no difference in single quotes or double quotes for the string creation.
Python programming language does not support a separate character (char) data type to hold single character so a Python string data type with a single character assigned to it can be used for char type in Python
You can also explicitly set the data type of the variable to string when you are assigning value to a variable using the str() function as shown in the code below
message = str("1.2")
How to Assign String to a Variable in Python
In Python programming language you can assign a string to a variable by following the variable name with an equal sign and the sequence of characters in single or double quotes. You can even make use of the str() function after the equal sign.
Here is the source code to demonstrate how to assign a string to a variable in Python
var1 = "Hello, World!" var2 = str("Hello, World!") print(var1) print(var2) print(type(var1)) print(type(var2))
Below is the output of the above code
Hello, World!
Hello, World!
<class 'str'>
<class 'str'>
You can see from the above output that both the variables var1 and var2 are assigned the string values.
How to Assign a Multiline String to a Variable in Python
In Python programming language triple quotes can be used to assign a multiline string to a variable in Python.
Here is the source code to demonstrate how to assign a multiline string to a variable in Python
var1 = '''Hello, World!''' print(var1) print(type(var1))
Below is the output of the above code
Hello,
World!
<class 'str'>
You can see from the above output that the variable var1 is assigned with a multiline string value.
What is Python String Index
In Python programming language string is encoded as ASCII or Unicode characters. So in a way, Python strings are a sequence of Unicode characters. This sequence is like an array starting with index 0. Each character in the Python string can be accessed using these indexes.
Here is the source code to demonstrate what is Python string index
var1 = 'Hello, World!' print(var1[0]) print(var1[1]) print(var1[2]) print(var1[3]) print(var1[4])
Below is the output of the above code
H e l l o
As you can see from the above output we are able to access the characters in the string using the index starting from 0.
How to access Python Strings as Arrays
In Python programming language string is a sequence of characters. This sequence of characters is like an array so we can loop through the characters in a string using Python Loops.
Here is the source code to demonstrate how to access Python strings as arrays
for x in "Orchid": print(x)
Below is the output of the above code
O
r
c
h
i
d
As we can see from the above output we are able to loop through the characters in a string using Python for loop.
How to access Elements of Python String using Index
Since Python string is encoded as Unicode characters and each character can be accessed using the string indexes. So we can even loop through the strings as a sequence of characters with indexes using the Python while loop as shown in the below code
Here is the source code to demonstrate how to access elements of Python String using the Index
var1 = 'Hello, World!' count = 0 while count < len(var1): print(var1[count]) count += 1
Below is the output of the above code
H
e
l
l
o
,
W
o
r
l
d
!
As you can see from the above output we were able to access each character in the string by using the string indexes with a Python while loop.
How to determine the Length of a String in Python
In Python programming language we can make use of the len() function to determine the number of characters in a string i.e. length of a string in Python.
Here is the source code to demonstrate how to determine the length of a string in Python
var1 = 'Hello, World!' print(len(var1))
Below is the output of the above code
13
As you can see from the above output making use of the len() function we got the number of characters in the string.
How to Modify a String in Python
In Python programming language we can modify a string in Python by re-assigning the variable a new string value. It is not possible to partially modify a string instead you have to assign it a new string value altogether.
Here is the source code to demonstrate how to modify a string in Python
var1 = 'Hello, World!' print(var1) var1 = 'Hello, Code Handbook!' print(var1)
Below is the output of the above code
Hello, World!
Hello, Code Handbook!
As you can see from the above output we were able to modify a string in Python by re-assigning the variable var1 to a new string value altogether.
How to Delete a String in Python
In Python programming language we can delete a string in Python by making use of the del keyword. The del keyword in Python is used to delete objects. In Python string is an object so the del keyword can be used to delete a string. We cannot delete a partial string in Python but we can delete the entire string in Python
Here is the source code to demonstrate how to delete a string in Python
var1 = 'Hello, World!' print(var1) del var1 print(var1)
Below is the output of the above code
Hello, World!
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'var1' is not defined. Did you mean: 'vars'?
As you can see from the above result that the del keyword against variable var1 has deleted the string variable var1.
How to Check if a Python String Contains Specified Characters
In Python programming language we can check if a string contains specified characters or not i.e. we can check if a certain phrase or characters are present in the specified Python string or not.
Here is the source code to demonstrate how to check if a Python string contains specified characters
message = "Hello, World!" print("World" in message)
Below is the output of the above code
True
As we can see from the above output that the code returns True as the phrase “World” is present in the specified string variable message.
We can also make use of the if statement to check if the specified phrase exists in the string or not. Below is the sample code using the if statement to check if Python String Contains specified Characters
message = "Hello, World!" if "World" in message: print("Yes, World Exists in message")
Below is the output of the above code
Yes, World Exists in message
As we can see from the above output that the code prints the string specified in the print statement as the phrase “World” is present in the specified string variable message.
How to Check if Python String Does not Contain a Specified Phrase
In Python programming language we can check if a string does not contain the specified characters i.e. we can check if a certain phrase or character does not exist in the Python string.
Here is the source code to demonstrate how to check if a Python string does not contain a specified phrase
message = "Hello, World!" print("handbook" not in message)
Below is the output of the above code
True
As we can see from the above output that the code returns True as the phrase “handbook” is not present in the specified string variable message.
We can also make use of the if statement to check if the specified phrase does not exist in the string. Below is the sample code using the if statement to check if Python String does not contain specified characters
message = "Hello, World!" if "handbook" not in message: print("No, handbook does not Exists in message")
Below is the output of the above code
No, handbook does not Exists in message
As we can see from the above output that the code prints the string specified in the print statement as the phrase “handbook” is not present in the specified string variable message.
Summary
In Python programming language String is a sequence of characters that are represented by either single or double quotes and the length can be determined for a Python string. In Python, strings can be either single or multiline and can even be created, modified and deleted. In Python, strings can also be accessed as an array using the index.
You can also check my series on Python for Beginners – Series: Python Tutorial – Learn Python Programming for Beginners
References – Python Documentation
Please provide your suggestions & feedback in the comments section below
Hope you found this article useful. Please support the Author
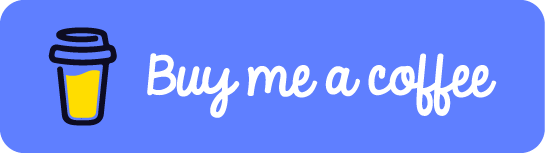