Let’s learn about how to use Python while loop. The Python while loop like any other language while loop can be used to execute the set of statements as long as the condition specified in the while loop is true. This can be any condition that returns a boolean value i.e. either true or false.
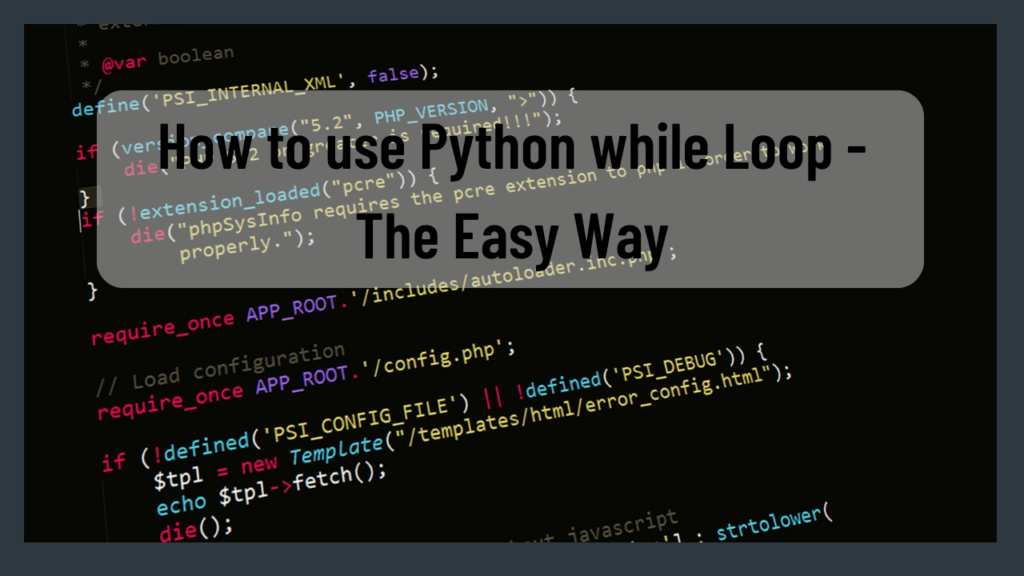
Using the while loop, we can write a set of code statements that will be executed until the condition specified in the Python while loop is true.
Here is the code syntax for how to use Python while Loop
count = 1 while count < 5: print(count) count += 1
Below is the output of the above code
1
2
3
4
As you can see from the above output the statements in the Python while loop has executed till the condition (count <5) specified in the while loop is true. After the count has reached a value of 5 the Python while loop stopped its execution.
In a while loop always remember to make changes in the while loop condition else it will turn out to be an infinite while loop. As in the above code if you forget to increment the count variable then it will always remain less than 5 and while loop will not end.
Use of break statement in Python while Loop
The break statement is used to stop the execution of the while loop even if the while loop condition is still true.
Now this stoppage of the while loop in between can be based on some other condition and if that condition is matched then stop or break the python while loop.
Here is the code syntax to demonstrate the use of the break statement in Python while Loop
count = 1 while count < 5: print(count) if count == 3: break count += 1
Below is the output of the above code
1
2
3
As you can see from the above output that after the count has been incremented to value 3 the while loop has stopped its execution due to a break statement based on the condition that the count is equal to 3.
Use of Else statement in Python while Loop
The else statement is used to specify the code statements that should be executed after the conditions of the while loop have turned false.
When you have a requirement like you need to run a set of code statements after the while loop condition is no longer true in that case you can use the Else statement in Python while loop.
Here is the source code to demonstrate how to use the Else function in Python while Loop
count = 1 while count < 5: print(count) count += 1 else: print("Finally count is not less than 5")
Below is the output of the above code
1
2
3
4
Finally count is not less than 5
As you can see from the above output the code in the Else part of the python while loop has executed after the condition of the python while loop has turned false i.e. value of count is 5 means count is no longer less than 5
Use of continue statement in Python while loop
The continue statement is used to stop or skip the execution of the current iteration and continue the execution of the while loop with the next iteration.
If there is a requirement like you need to process all the iterations in the Python while loop except for some specific iteration based on some condition, in that case, you can use the continue statement to skip the execution of the Python while loop for that specific iteration.
Here is the source code to demonstrate the use of the continue statement in Python while Loop
count = 0 while count < 5: count += 1 if count == 2: continue print(count)
Below is the output of the above code
1
3
4
5
As you can see from the above output that the Python while loop processing for the count value 2 has been skipped as we have specified the continue statement for count value 2 and all other iterations before and after count value 2 have been processed.
Summary
In this article, we learnt about the while loop in Python and we also explored how to use various features of the while loop in the Python programming language. The while loop is a very popular and widely used loop not only in Python but in other languages as well.
You can also check my series on Python for Beginners – Series: Python Tutorial – Learn Python Programming for Beginners
References – Python Documentation
Please provide your suggestions & feedback in the comments section below
Hope you found this article useful. Please support the Author
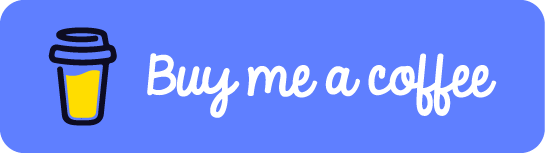