In this article, we will learn about loops and conditionals in Python. We will learn about for loop, while loop and if statement that helps us control the flow of the program by repeating the same set of code statements or running different code logic based on conditions.
The loops and conditionals are very important and essential in any programming language including Python as it helps to control the flow of a program. Python also supports different ways of implementing loops & conditionals in Python.
Loops allow developers to write the code statements once and repeat either a fixed number of times or a variable number of times which is based on some conditions. Loop also help to reduce the lines of code as there is no need to repeat the code to run it again.
The if statement can be used to make decisions in the code. i.e. if you have a situation where you need to run different code statements based on some condition then if statement can be used for the same.
This is the seventh article in the Series: Python Tutorial – Learn Python Programming for Beginners
So far as part of this series we have looked at the basics of Python Programming along with the installation of Python & Visual Studio Code as IDE for Python, variables in Python and data types in Python.
Now let’s learn about Loops & Conditionals in Python which will help us understand how to build the required logic or algorithm using loops like For/While Loop & if statements.
Table of Contents
Loops and Conditionals in Python
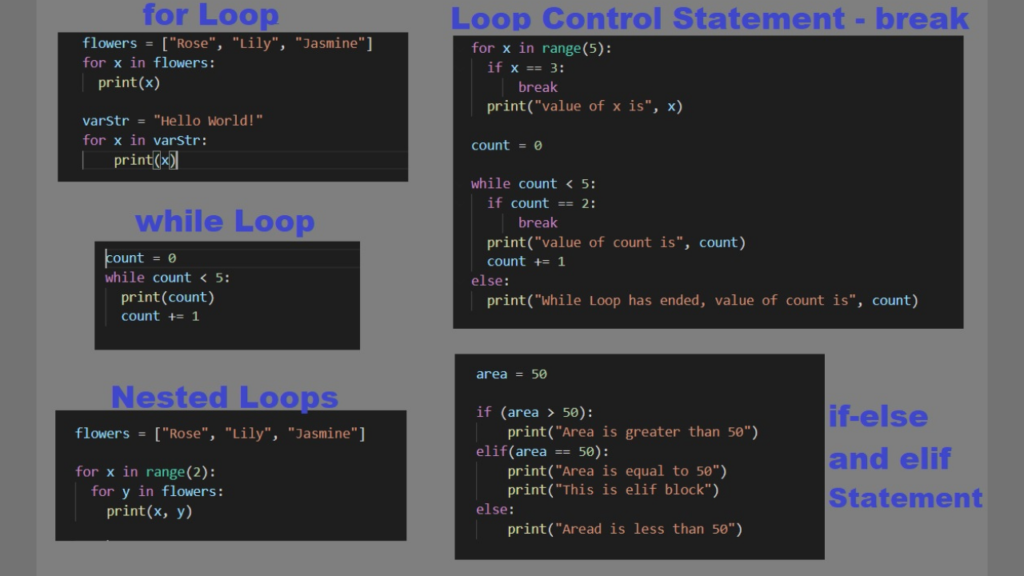
In general, in any programming language statements are executed sequentially i.e. in the order in which they are written. Now if you want some control over this flow i.e. either you want to repeat one or more lines of code or skip some lines of code based on some condition then what we will need is loops and conditionals in Python to control the flow of the code.
So let’s look at the detailed syntax of how For Loop, While Loop & if statements. Also, let’s see how and where to use these loops & if statements.
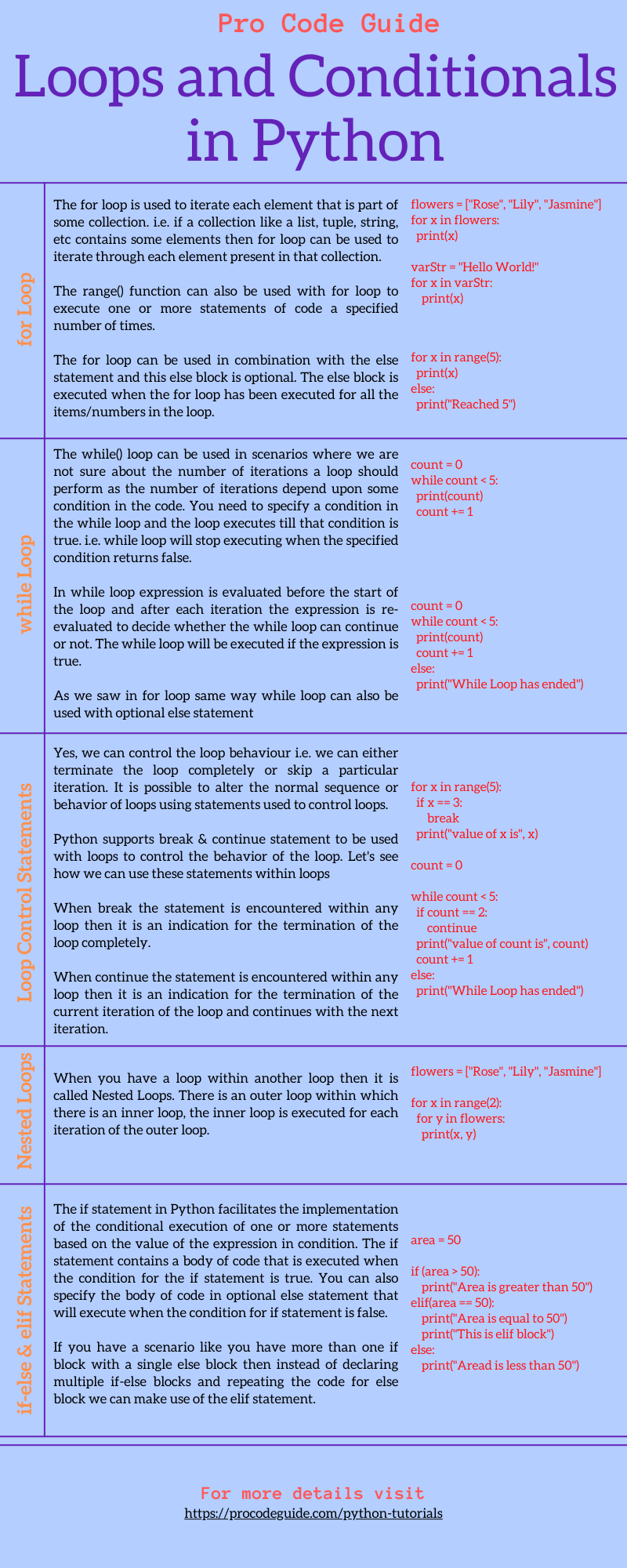
for Loop
The for loop is mostly used in the place where we already know the number of times we need to iterate over one or more lines of code. Yes, while loop also can be used in this scenario but for loop is a better implementation for definitive iteration scenarios.
The for loop is used to iterate each element that is part of some collection. i.e. if a collection like a list, tuple, string, etc contains some elements then for loop can be used to iterate through each element present in that collection. The one or more lines of code that are part of for loop & need to be repeated will be repeated once for each element in the collection. In fact, for loop can be used to iterate any iterable object in Python.
Below are a couple of examples that show how to iterate through the sequence of characters in the string and iterate each item in the list using for loop. The code in the for loop uses indentation to separate itself from the rest of the code.
flowers = ["Rose", "Lily", "Jasmine"] for x in flowers: print(x) varStr = "Hello World!" for x in varStr: print(x)
Below is the output of the above code where for loop iteration for each item in flowers and for each character in “Hello World!”
Rose
Lily
Jasmine
H
e
l
l
o
W
o
r
l
d
!
Using for Loop with range() Function
The use of the range() function with for loop is to execute one or more statements of code a specified number of times. The range() function can be used to set the number of times in the for a loop. The definition of range function is range(start, stop, step_size)
The range() functions return a sequence of numbers based on the input to the range function. This sequence of numbers by default starts from 0 and increments the number by 1 each time. Below is the sample code of range() function with for loop where we are requesting numbers from range function as a range(5) so range function will return 5 numbers starting from 0 and incrementing by 1. This number 5 specifies where should the loop end i.e. no numbers beyond 5 and it doesn’t include number 5 as well.
for x in range(5): print(x)
As seen below the output of the above range(5) function is returning 5 number starting from 0 to 4 & it doesn’t include 5 & also doesn’t goes beyond 5
0
1
2
3
4
Let’s change the input to the range function to return numbers starting from 2 instead of default 0 and numbers till 6 instead of 5. We have not set the default increment value which is 1
for x in range(2, 6): print(x)
As seen in the below output of the above code, the range function is returning numbers starting from 2 (instead of default 0) till 5 (doesn’t include 6)
2
3
4
5
Now let’s check the output of the range function when you specify the increment value. In the below code, we have set increment value as 2 for range function where we have specified it to start numbers from 2 and return till 11 number
for x in range(2, 11, 2): print(x)
Below is the output of the above range function where we can see that numbers are starting from 2, incrementing step is 2 and returned numbers till 10
2
4
6
8
10
So far we have learned about how to use for loop with collections like list, and string & also in combination with range function. It is also possible to use for loop with an else statement so let’s now learn about how and where to use for loop with an else statement.
Using for Loop with else Statement
The for loop can be used in combination with the else statement and this else block is optional. The else block is executed when the for loop has been executed for all the items/numbers in the loop.
Now if you exit or stop the for loop in between then else block is never executed. Below is the code of the else block in for loop where we are using the range() function in for loop for 5 numbers and also specified an else block.
for x in range(5): print(x) else: print("Reached 5")
After running the above code we get the below output where we can see that for loop has executed completely for range function and then after printing all numbers returned by range function it has executed else block to print ‘Reached 5’
0
1
2
3
4
Reached 5
while Loop
The while() loop can be used in scenarios where we are not sure about the number of iterations a loop should perform as the number of iterations depends upon some condition in the code. You need to specify a condition in the while loop and the loop executes till that condition is true. i.e. while loop will stop executing when the specified condition returns false.
In a way, we can even say that while loop is a repeating if statement where one or more lines of code get repeated based on some expression.
You need to be careful with while loops as if you don’t use your condition properly i.e. if there is a mistake in code/logic due to which condition never becomes false then it will turn into an infinite loop that never ends.
In while loop expression is evaluated before the start of the loop and after each iteration the expression is re-evaluated to decide whether the while loop can continue or not. The while loop will be executed if the expression is true.
The code in the while loop uses indentation to separate itself from the rest of the code. Below is the code sample for the while loop.
count = 0 while count < 5: print(count) count += 1
When we execute the above code we get the results as shown below. We can see from the below output that while loop executes till the condition is true i.e. value of count should be less than 5
0
1
2
3
4
Don’t forget to change the value of count i.e. increment count else it will become an infinite while loop.
Using while loop with else statement
As we saw in for loop same way while loop can also be used with optional else statement. When the execution of the while loop completes with the condition for the while loop becoming false then the statement in the else block will be executed.
Now if you exit or stop the while loop in between then else block is never executed. Below is the sample code of the while loop with the else statement
count = 0 while count < 5: print(count) count += 1 else: print("While Loop has ended, value of count is", count)
Below is the output of the above code. We can see that the print statement is executed in the else statement when while loop has ended i.e. the value of the count is not less than 5.
0
1
2
3
4
While Loop has ended, value of count is 5
Controlling Loop Behavior
Yes, we can control the loop behaviour i.e. we can either terminate the loop completely or skip a particular iteration. It is possible to alter the normal sequence or behaviour of loops using statements used to control loops.
Python supports break
& continue
statement to be used with loops to control the behaviour of the loop. Let’s see how we can use these statements within loops
Break Statement
When a break
statement is encountered within any loop then it is an indication for the termination of the loop completely. On encountering a break statement control of the code execution is transferred to the first line outside of the loop. In the case of a while loop also the execution is terminated even if the expression for while is true.
Below is the code with the usage of the break statement in for and while loop. In the code below the break, statement is used to abort the loop based on some condition in both for and while loops.
for x in range(5): if x == 3: break print("value of x is", x) count = 0 while count < 5: if count == 2: break print("value of count is", count) count += 1 else: print("While Loop has ended, value of count is", count)
When we execute the above code we get the below output where we can see that loop is totally stopped when a break statement is encountered during the execution of that loop.
value of x is 0
value of x is 1
value of x is 2
value of count is 0
value of count is 1
One more point to note is that when the loop is terminated using a break statement then the else statement for the loop is also not executed as the loop has not ended but has been stopped in between.
Continue Statement
When continue
statement is encountered within any loop then it is an indication for the termination of the current iteration of the loop and continues with the next iteration. On encountering a continue statement control of the code execution is transferred to the expression of the loop to evaluate if the loop can continue and if yes then the loop is executed for the next iteration.
Below is the code with the usage of the continue statement in for and while loop. In the code below continue statement is used to abort the current iteration based on some condition in both for and while loops.
for x in range(5): if x == 3: continue print("value of x is", x) count = 0 while count < 5: if count == 2: count += 1 continue print("value of count is", count) count += 1 else: print("While Loop has ended, value of count is", count)
When we execute the above code we get the below output where we can see that loop iteration is skipped when a continue statement is encountered during the execution of that iteration in the loop.
value of x is 0
value of x is 1
value of x is 2
value of x is 4
value of count is 0
value of count is 1
value of count is 3
value of count is 4
While Loop has ended, value of count is 5
There is no impact on exit statements with the usage of the continue statement in the loops.
Nested Loops
When you have a loop within another loop then it is called Nested Loops. There is an outer loop within which there is an inner loop, the inner loop is executed for each iteration of the outer loop.
Below is the sample code for nested loop where we have written for loop with range and within that, for loop, we have added another for loop to print flowers name
flowers = ["Rose", "Lily", "Jasmine"] for x in range(2): for y in flowers: print(x, y)
Below is the output of the above code where we can see that the inner loop for flower name print has been executed for each iteration of the for loop with a range
0 Rose
0 Lily
0 Jasmine
1 Rose
1 Lily
1 Jasmine
if-else Statement
While writing programs many times we come across situations like we need to run a block of code based on some conditions. For example, one very simple scenario can be like we need to calculate either simple interest or compound interest based on the input. So making a decision based on some condition is one of the most important aspects of any programming language.
The if statement in Python facilitates the implementation of the conditional execution of one or more statements based on the value of the expression in condition. The if statement contains a body of code that is executed when the condition for the if statement is true. You can also specify the body of code in the optional else statement that will execute when the condition for the if statement is false.
Below is the sample syntax/usage of the if-else statement in Python. The code in the if-else statement uses indentation to separate itself from the rest of the code.
if (condition):
# This block executes when condition is true
else:
# This block executes when condition is false
Below is the sample code which demonstrates the if-else statement in Python. We have declared the variable area and set it to a value of 100. Using the if-else statement we have set blocks like area greater than 50 and the area equal to or less than 50.
area = 100 if (area > 50): print("Area is greater than 50") else: print("Aread is less than 50")
We get the below output when we run the above code. As expected we get output from the block area greater than 50 as the area is set to 100
Area is greater than 50
elif statement
If you have a scenario where you have more than one if block with a single else block then instead of declaring multiple if-else blocks and repeating the code for the else block we can make use of the elif statement as shown in the below code.
area = 50 if (area > 50): print("Area is greater than 50") elif(area == 50): print("Area is equal to 50") print("This is elif block") else: print("Aread is less than 50")
We get the below output for the above code. We have used the if statement to check the condition for an area greater than 50, then we have made use of the elif statement to check the condition for an area equal to 50 and finally an else statement for a condition area less than 50.
Area is equal to 50
This is elif block
We learned about syntax and usage of if-else and elif statements as part of loops and conditionals in Python.
Summary
In this article, we learned about for loop, while loop, loop control statements, nested loops and if statements as part of loops & conditionals in Python. This will help you to build your program logic as per the requirements with the option to repeat statements conditionally using loops and/or running different blocks of statements based on conditions on the if statement in Python.
In our next article, we will learn about Collection and Mapping Types in the Python programming language.
Please provide your suggestions & questions in the comments section below
References – Sequence Types in Python
Hope you found this article useful. Please support the Author
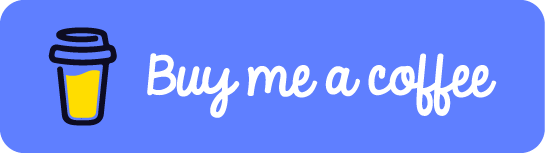