This article will teach us about Python For Loop with easy examples. We will also learn about the various operations we can perform with the For loop in Python.
In software programming languages, loops are used to repeat a block of code. These number of repetitions are based on some condition which can be specified and also the number of repetitions can be controlled by using various statements like a break or continue that can be used in conjunction with the for loop.
So let’s dive deeper into How to use Python For Loop
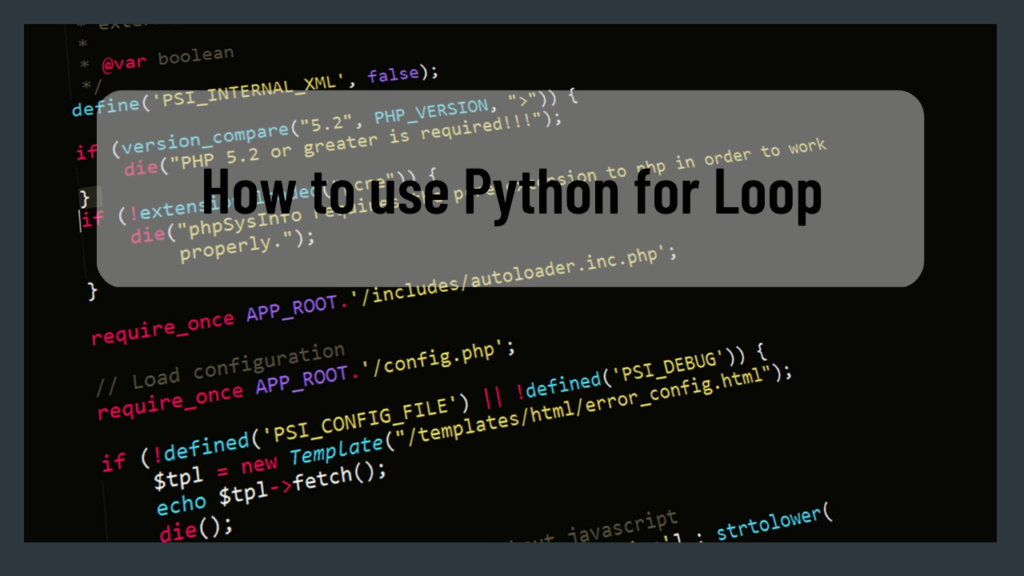
Python for loop, like any other language, can be used to iterate or traverse through a sequence of items. These items can be a list, tuple, dictionary, string or set.
Using the for loop we can write a set of statements that will be executed for each item in the sequence.
Here is the solution for how to use Python for Loop
flowers = ["Rose", "Lily", "Jasmine"] for x in flowers: print(x)
Below is the output of the above code
Rose
Lily
Jasmine
for loop to loop through a String in Python
The string is a sequence of characters and the string is also an iterative object. You can iterate a string in Python using for loop
Here is the source to loop through a String using for loop in Python
for x in "Orchid": print(x)
Below is the output of the above code
O
r
c
h
i
d
Let’s look at the source code to loop a string that is available in a variable.
flower = "Lily" for x in flower: print(x)
Below is the output of the above code
L
i
l
y
Use of break statement in Python for Loop
The break statement is used to stop the execution of the loop even before it has iterated all the items in the sequence.
Now this stoppage of the loop in between can be based on some condition i.e. if the value of the item is equal to something then stop or break the for loop. The condition can even be like if python for loop has executed n number of times then stop the execution of the loop.
Here is the source to demonstrate the use of break statements in Python for Loop
flowers = ["Lily", "Orchid", "Tulip", "Lavender"] for x in flowers: print(x) if x == "Tulip": break
Below is the output of the above code
Lily
Orchid
Tulip
As you can see from the above output that after item Tulip the for loop has stopped execution due to a break statement and so item Lavender was not processed.
Use of the continue statement in Python for loop
The continue statement is used to stop the execution of the current item or iteration in the sequence and continue with the for loop with the remaining items in the sequence.
If there is a requirement like you need to process all the items in the sequence except some specific item then in that case we can skip the execution of for loop statement for that particular item in the sequence.
Here is the source to demonstrate the use of the continue statement in Python for Loop
flowers = ["Lily", "Orchid", "Tulip", "Lavender"] for x in flowers: if x == "Tulip": continue print(x)
Below is the output of the above code
Lily
Orchid
Lavender
As you can see from the above output for the item Tulip in the list of flowers the for loop didn’t get processed due to the continue statement and so the item Tulip was not processed i.e. not printed.
Use of the range function in Python for Loop
The range function is used to return a sequence of numbers and by default, this number sequence starts at 0 & the end number depends on the number specified in the range function.
If you have a requirement like you need to implement a for loop that executes the specified statements a fixed number of times, then you can use the range function in the Python for loop. Through code syntax let’s check how to use the range function in Python for loop
Here is the source code to demonstrate how to use the range function in Python for Loop
for x in range(6): print(x)
Below is the output of the above code
0
1
2
3
4
As you can see from the above output for range(5) the numbers returns are 0 to 4. i.e. its starts at 0 and return the sequence of numbers till 4 as number 5 is specified in the range function.
As you can see range function by default starts at 0 but It is also possible to change this default to 0 zero by specifying the number at which you want that the range returned number should start from. Let’s check how to specify the start of the number in the range function.
for x in range(2, 6): print(x)
Below is the output of the above code
2
3
4
5
You can see in the above code that range(2, 6) sets the starting number at 2 and ends at 5 (does not end at 6).
As you can see range function by default increments the number by 1 but It is also possible to change this default increment of 1 by specifying the number by which you want that the range returned number should increment by what number. Let’s check how to specify the increment number in the range function.
for x in range(2, 10, 2): print(x)
Below is the output of the above code
2
4
6
8
You can see in the above code that range(2, 10, 2) sets the starting number at 2, ends at 8 (does not end at 10) and increments the number by 2 instead of default 1.
So by using the range function in the for loop you can iterate the for loop a fixed number of times and also control the sequence of numbers returned by the range function.
Use of the Else statement in Python for Loop
The else statement is used to specify the code statements that should be executed after the for loop has finished execution of all the items in the defined iteration.
When you have a requirement like you need to run a set of code after the for loop has ended then in that case you can use the Else statement in Python for loop.
Here is the source code to demonstrate how to use the Else function in Python for Loop
flowers = ["Rose", "Lily", "Jasmine"] for x in flowers: print(x) else: print("All Flowers Processed")
Below is the output of the above code
Rose
Lily
Jasmine
All Flowers Processed
As you can see from the above output the code in the Else part of the python for loop has executed after the for loop has finished processing for all the flowers in the list.
Implement Empty for Loop in Python
The pass statement is used to implement a for loop in python that does not do anything during each iteration.
It is not possible to implement a for loop in python with no contents but if you have a requirement to implement an empty for loop then, in that case, you can make use of the pass statement in for loop to implement an empty for loop in python.
Here is the source code to demonstrate how to implement Empty for Loop in Python
for x in [0, 1, 2]: pass
Below is the output of the above code
As you can see above that there is no output for the code as it is empty for loop in Python i.e. Python for loop with no contents.
Implement Nested for Loop in Python
The nested for loop is a for loop within another for loop in Python.
When you have a requirement like you need to call a for loop within another loop then in that case you can make use of a nested for loop in Python.
Here is the source code to demonstrate the syntax for Nested for Loop in Python.
colors = ["Red", "Blue", "White", "Gray"] cars = ["BMW", "Audi", "Mercedes"] for color in colors: for car in cars: print(color, car)
Below is the output of the above code
Red BMW
Red Audi
Red Mercedes
Blue BMW
Blue Audi
Blue Mercedes
White BMW
White Audi
White Mercedes
Gray BMW
Gray Audi
Gray Mercedes
As you can see from the above output the inner cars loop has iterated for all the values of cars for each value of the outer For loop for colours.
Summary
In this article, we learnt about Python for loop and we also explored how to use various features of for loop in the Python programming language. The for loop is a very popular and widely used loop not only in Python but in other languages as well.
You can also check my series on Python for Beginners – Series: Python Tutorial – Learn Python Programming for Beginners
References – Python Documentation
Please provide your suggestions & feedback in the comments section below
Hope you found this article useful. Please support the Author
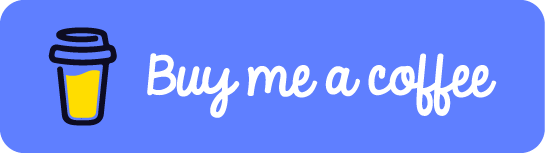