Polymorphism is one of the important building blocks in object-oriented programming techniques. Polymorphism is a greek word that means “many-shaped” and also Poly means many whereas morph means forms i.e. many forms of an object.
We can say that polymorphism is an ability of an object to be projected in more than one form or we can say that it is a concept using which the same action can be performed in different forms.
Introduction to Polymorphism
Polymorphism means design by interface i.e. many different classes implement the same interface to provide the same interface methods but each class has its own implementation of those methods.
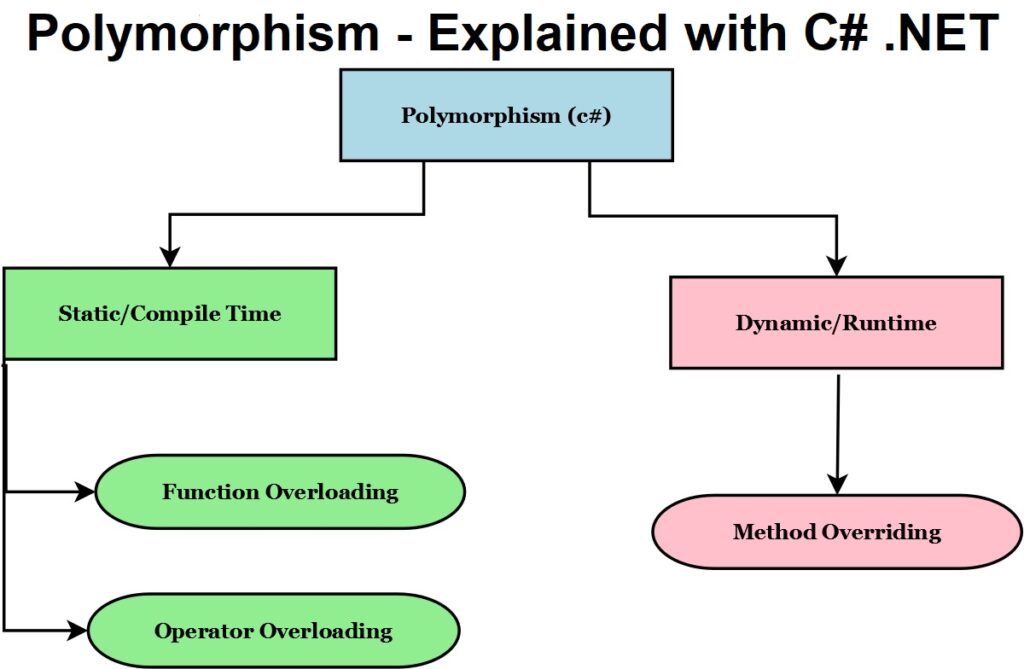
Polymorphism occurs when a parent class reference is used to refer to an instance of a child class object. An object is said to be polymorphic when it passes more than one IS-A test. As in C#, the Object class is the base class of the classes so any object at any given time will be polymorphic as it passes the IS-A test for its own type and as well as to object type.
The class can be accessed through its instance set to a reference variable. A reference variable is always of one single type which cannot be changed. A reference variable can be set to an instance of declared type i.e class or any other class derived from this class.
Types of Polymorphism
There are two types of polymorphism in c# .NET
- Static/Compile time
- Dynamic/Runtime
Static/compile time is achieved by method overloading or operator overloading & dynamic/Runtime is achieved by method overriding.
Function Overloading – It is a feature in c# .NET where two or more functions can have the same name but different parameters. Due to the different sets of parameters, each function has a different signature.
Operator Overloading – It is a feature in c# .NET that allows the same operator to do various operations i.e. it allows to provide new definitions to the given operator.
Function Overriding – It is a feature in c# .NET which allows a child class to have a function that is already present in the base class. The child class can have its own implementation of the overridden function.
Polymorphism with C# .NET example
The below code shows an example of function overloading. There is a logger class with multiple implementations of the Log function either to log an error message or exception object or both. Based on arguments passed by the calling code appropriate implementation of the function will be called.
class Logger { public void Log(string message) { //Write Message To Log return; } public void Log(Exception ex) { //Write Exception To Log return; } public void Log(string message, Exception ex) { //Write Message & Exception To Log return; } }
The below code shows an example of operator overloading. + operator is overloaded to add two classes of type room and return details like total length and breadth.
class Room { private double length; private double breadth; public double Length { get { return length; } set { length = value; } } public double Breadth { get { return breadth; } set { breadth = value; } } public double GetArea() { return (length * breadth); } public static Room operator+ (Room room1, Room room2) { Room room = new Room(); room.Length = room1.Length + room2.Length; room.Breadth = room1.Breadth + room2.Breadth; return room; } }
The below code shows an example of dynamic polymorphism. The base class is MotorVehicle & child classes MotorCycle & MotorCar are derived from MotorVehicle. The base class has the virtual function GetNumberOfWheels & this virtual keyword will force child classes to override this function and provide their own implementation.
Here reference variable motorVehicle is of type MotorVehicle but it can be set to an instance of all 3 classes MotoVehicle, MotorCycle & MotorCar as it passes the IS-A test for all 3 classes. When the same reference variable is set to a different instance then the result of function GetNumberOfWheels is also different i.e. implementation of set instance is called.
class MotorVehicle { public virtual string GetNumberOfWheels() { return "Motor Vehicle has two or more wheels"; } } class MotorCycle : MotorVehicle { public override string GetNumberOfWheels() { return "Motor Cycle has two wheels"; } } class MotorCar : MotorVehicle { public override string GetNumberOfWheels() { return "Motor Car has four wheels"; } } //Client Code MotorVehicle motorVehicle = new MotorVehicle(); MessageBox.Show(motorVehicle.GetNumberOfWheels()); //Motor Vehicle has two or more wheels motorVehicle = new MotorCycle(); MessageBox.Show(motorVehicle.GetNumberOfWheels()); //Motor Cycle has two wheels motorVehicle = new MotorCar(); MessageBox.Show(motorVehicle.GetNumberOfWheels()); //Motor Car has four wheels
The below code shows the implementation of dynamic polymorphism by using Interface.
interface MotorVehicle { string GetNumberOfWheels(); } class MotorCycle : MotorVehicle { public string GetNumberOfWheels() { return "Motor Cycle has two wheels"; } } class MotorCar : MotorVehicle { public string GetNumberOfWheels() { return "Motor Car has four wheels"; } } //Client Code MotorVehicle motorVehicle = null; motorVehicle = new MotorCycle(); MessageBox.Show(motorVehicle.GetNumberOfWheels()); //Motor Cycle has two wheels motorVehicle = new MotorCar(); MessageBox.Show(motorVehicle.GetNumberOfWheels()); //Motor Car has four wheels
Summary
Polymorphism is a fundamental block of object-oriented programming & it helps to process objects of various types through a single interface.
Static or compile-time polymorphism is achieved by method or operator overloading and dynamic or Runtime polymorphism is achieved by method overriding are two types of polymorphism supported by c# .NET
In c# all objects are polymorphic as all classes have default base class objects.
Please provide your suggestions & questions in the comments section below
Reference: https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/classes-and-structs/polymorphism
Check my other article related to OOP concepts – https://procodeguide.com/programming/oop-concept-encapsulation-in-c-net/
You can also check my other trending articles on .NET Core to learn more about developing .NET Core Applications
- Microsoft Feature Management – Feature Flags in ASP.NET Core C# – Detailed Guide
- Microservices with ASP.NET Core 3.1 – Ultimate Detailed Guide
- Entity Framework Core in ASP.NET Core 3.1 – Getting Started
- Series: ASP.NET Core Security – Ultimate Guide
- ML.NET – Machine Learning with .NET Core – Beginner’s Guide
- Real-time Web Applications with SignalR in ASP.NET Core 3.1
- Repository Pattern in ASP.NET Core with Adapter Pattern
- Creating an Async Web API with ASP.NET Core – Detailed Guide
- Build Resilient Microservices (Web API) using Polly in ASP.NET Core
Hope you found this article useful. Please support the Author
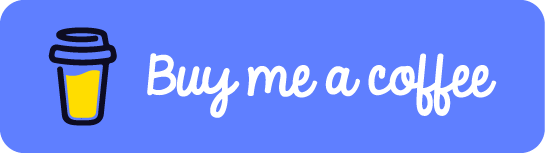